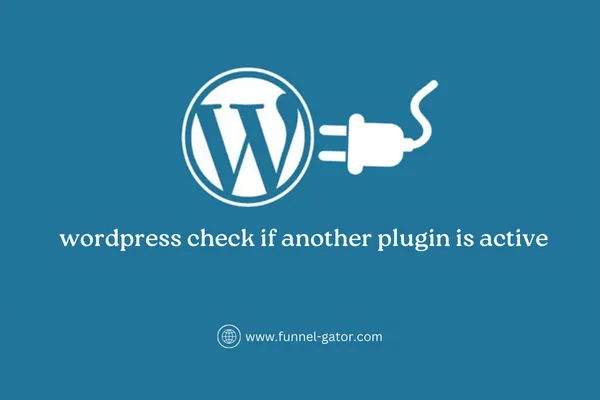
WordPress Check if Another Plugin is Active: A Complete Guide
Managing plugins in WordPress can sometimes be tricky, especially when you need to ensure plugin compatibility or determine whether a specific plugin is active before executing code. In this guide, we’ll cover how to check if another plugin is active, why it’s important, and the best ways to troubleshoot conflicts.
Why Check If Another Plugin is Active?
Checking if a plugin is active is crucial in several situations, such as:
✔️ Preventing conflicts between multiple plugins that perform similar tasks.
✔️ Enhancing compatibility by ensuring required plugins are installed.
✔️ Avoiding errors when a dependent plugin is missing or inactive.
✔️ Customizing functionality based on active plugins.
For example, if you’re building a custom theme or plugin that relies on WooCommerce, you need to check if WooCommerce is active before using its functions. Otherwise, you might run into fatal errors.
Methods to Check If a WordPress Plugin is Active
There are several ways to check if a plugin is active, ranging from code snippets to using plugin checkers. Let’s explore each method.
1. Using PHP Code in Your Theme or Plugin
WordPress provides built-in functions to check if a plugin is active. The most common one is:
Method 1: Using is_plugin_active()
The is_plugin_active()
function checks whether a specific plugin is active in the WordPress installation.
php
if (is_plugin_active('woocommerce/woocommerce.php')) {
echo 'WooCommerce is active!';
}
🔹 How it works:
The function checks for
woocommerce/woocommerce.php
in the plugins directory.If the plugin is active, the condition returns true, and the message "WooCommerce is active!" is displayed.
🔹 Where to use it:
Inside a theme’s functions.php file.
Inside a custom plugin to ensure compatibility.
2. Using class_exists()
to Check for Plugin Classes
Some plugins define unique PHP classes when activated. Instead of checking the plugin folder name, you can check if the plugin’s main class exists:
php
if (class_exists('WooCommerce')) {
echo 'WooCommerce is installed and active!';
}
🔹 When to use this method:
When the plugin defines a specific class.
When the folder name might vary, but the class remains the same.
3. Checking Network-Activated Plugins in Multisite
If you’re using WordPress Multisite, plugins can be activated network-wide instead of per-site. Use is_plugin_active_for_network()
to check for network-wide activation:
php
if (is_plugin_active_for_network('woocommerce/woocommerce.php')) {
echo 'WooCommerce is network activated!';
}
🔹 When to use this method:
When working with Multisite WordPress installations.
When ensuring a plugin is available across all sites.
4. Checking Active Plugins Using WP-CLI
If you have command-line access, you can use WP-CLI to list active plugins:
sh
wp plugin list --status=active
This will return a list of all active plugins, helping you identify any conflicting or missing plugins quickly.
Best Plugins to Check for Active Plugins and Conflicts
If you’re not comfortable editing code, you can use plugin compatibility checkers. Here are the best options:
1. Health Check & Troubleshooting
This free plugin allows you to troubleshoot plugin conflicts by disabling all plugins in a safe mode without affecting your live site
2. WP Hive Plugin Compatibility Checker
WP Hive analyzes plugin performance and compatibility before installation, helping you avoid conflicts
3. Better Plugin Compatibility Control
This tool checks plugin compatibility with WordPress versions, reducing the risk of issues after updates
4. Envato Tuts+ Plugin Detector
This tool scans active plugins on competitor websites, allowing you to see what plugins are running on any WordPress site
Troubleshooting Plugin Conflicts
If you suspect two plugins are conflicting, follow these steps to diagnose and resolve the issue:
Step 1: Deactivate All Plugins
Go to Plugins > Installed Plugins in the WordPress dashboard.
Deactivate all plugins except the one you’re testing.
Check if the issue persists.
Step 2: Reactivate Plugins One by One
Enable plugins one at a time.
Refresh the site after each activation to see if the issue returns.
Once the conflict appears, you’ve found the problematic plugin.
Step 3: Check Error Logs
Use the WP_DEBUG
mode in wp-config.php
to log errors:
php
define('WP_DEBUG_LOG', true);
define('WP_DEBUG_DISPLAY', false);
Step 4: Update Plugins and WordPress
Always update plugins to their latest versions.
Make sure WordPress core is up to date.
If an update causes issues, roll back to a previous version.
For a complete troubleshooting guide, check out WPMU DEV’s plugin conflict resolution
Final Thoughts
Checking whether a WordPress plugin is active is essential for preventing conflicts, optimizing performance, and ensuring compatibility. Whether you use PHP code, WP-CLI, or plugin checkers, knowing which plugins are running can save you time and prevent major website issues.
Key Takeaways
✔️ Use is_plugin_active()
to check plugin status in code.
✔️ Use class_exists()
for class-based plugin detection.
✔️ Use plugin checkers like WP Hive to analyze compatibility.
✔️ Troubleshoot plugin conflicts with Health Check & Troubleshooting.
✔️ Always update plugins and WordPress to avoid errors.
By following these methods, you can effectively manage plugins, avoid compatibility issues, and keep your WordPress site running smoothly.
Need help? Check out WPBeginner’s guide on plugin conflicts for more troubleshooting tips